Showing posts with label open cv. Show all posts
Saturday, 6 September 2014
Using opencsharp
Now this was much simpler than I thought, and since I don’t anticipate going into OpenCV functions to alter them, I will just run with the DLLs of OpenCV.
- download gitgui for windows. This program will allow us to easily clone GitHub repositories to our hard drive.
- clone the opencvsharp
- bottom-right corner→ press clone to desktop → put in a location (I chose C:/opencvsharp)
- compile and run some sample code
- open the solution at C:\opencvsharp\sample
- build all
- set a project as startup project (I chose CStyleSamples)
- run (F5)
You should get these outputs.
It works!!! If you are having problems, please post questions here.
Open CV setup
For all of us less fluent Visual Studio users, here are step by step of how I setup Visual Studio, Cmake, and OpenCV to running a working “hello world” type project. Please let me know if I’m doing something unconventional or that is bad practice. We’re all here to learn!
- install Visual Studio 2012 (or any other versions but do know which versions you are installing)
→ note: VC11 is Visual Studio 2012, VC12 is VIsual Studio 2013…
I installed it with all the standard settings, yes to everything
- install OpenCV latest version (I stay away from Alpha versions because they might have bugs and might not be supported by the latest OpenCVSharp which is my end goal.
http://sourceforge.net/projects/opencvlibrary/files/opencv-win/3.0.0-alpha/ → here I downloaded the OpenCV 2.4.9 version http://sourceforge.net/projects/opencvlibrary/files/latest/download?source=files
I went with all the default values and put it in the default directory “C:\opencv249”
- install cmake
go to this address
get the cmake-3.0.1-win32-x86.exe binaries, it makes your life way simpler because it just extracts itself. I went with default locations.
“C:\Program Files (x86)\CMake”
- write a .cpp file with enough to call some OpenCV functions (got this from the openCV site tutorials) http://docs.opencv.org/doc/tutorials/introduction/linux_gcc_cmake/linux_gcc_cmake.html#linux-gcc-usage
Put this file in this directory for example
.\Documents\Visual Studio 2012\Projects\DisplayImage
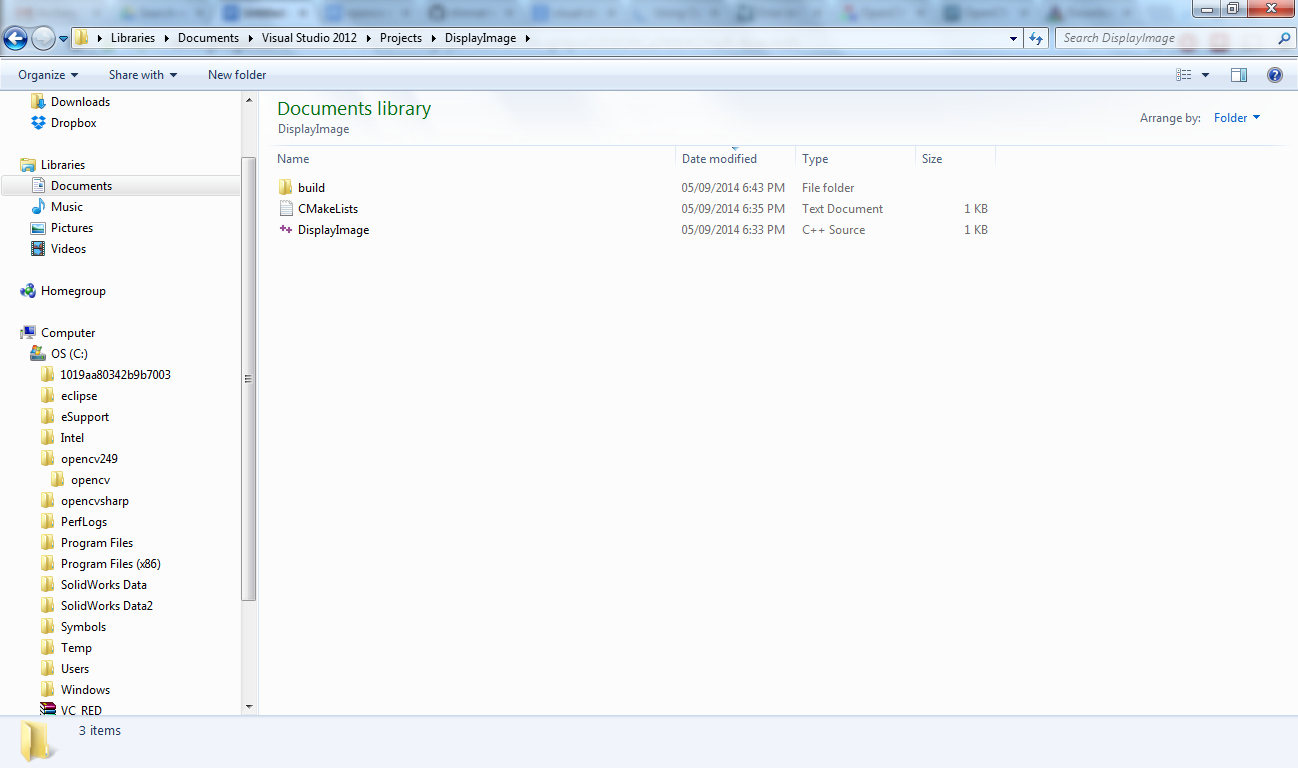
#include <stdio.h>
#include <opencv2/opencv.hpp>
using namespace cv;
int main(int argc, char** argv )
{
if ( argc != 2 )
{
printf("usage: DisplayImage.out <Image_Path>\n");
return -1;
}
Mat image;
image = imread( argv[1], 1 );
if ( !image.data )
{
printf("No image data \n");
return -1;
}
namedWindow("Display Image", CV_WINDOW_AUTOSIZE );
imshow("Display Image", image);
waitKey(0);
return 0;
}
- write a cmakelists.txt file that has this inside
cmake_minimum_required(VERSION 2.8)
project( DisplayImage )
find_package( OpenCV REQUIRED )
add_executable( DisplayImage DisplayImage.cpp )
target_link_libraries( DisplayImage ${OpenCV_LIBS} )
- run cmake.exe and browse to the location of the source file and cmakelists.txt
C:/Users/NAME/Documents/Visual Studio 2012/Projects/DisplayImage
point the build to where you want the solution and project files need to be.
C:/Users/NAME/Documents/Visual Studio 2012/Projects/DisplayImage/Build
- when prompted for which compiler, choose Visual Studio 2012 (I am using the 32bit version, you can tell it’s 32bits by the fact that the Visual Studio 2012 folder is under “c:/program files x86” folder.)
→ note: I had the error “error in configuration process” because I had selected Visual Studio 2013, selecting the right version should go through quite nicely
What’s happening here is that you are creating solution and project files that Visual Studio will be able to open, compile and build the project.
it will configure...
Then press Generate, it will generate
- Now go to “C:\Users\NAME\Documents\Visual Studio 2012\Projects\DisplayImage\build” where you just created the solution and project files.
Open the DisplayImage solution file
- Press F6 to build all.
- Now everything is built but this is a command line project so you need to run it from command line
- navigate to the build directory
C:\Users\NAME\Documents\Visual Studio 2012\Projects\DisplayImage\build\Debug
Bring in the needed dlls
→ opencv_highgui249d.dll
→ opencv_imgproc249d.dll
→ opencv_core249d.dll
Bring in an image of your choice (here I brought in an image I called lena.jpg)
(you can right-click and save this one as lena.jpg, to that build directory)
- Now navigate to the right directory in command prompt
type in DisplayImage lena.jpg and you will see the image you put in that directory!
Subscribe to:
Posts
(
Atom
)